Bubble Sort
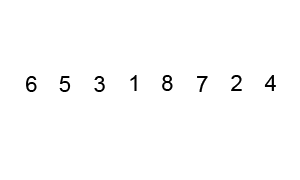
1 <?php 2 function swap(&$a, &$b){ 3 $c = $a; 4 $a = $b; 5 $b = $c; 6 } 7 8 # bubble sort 9 # ascend10 function sortBubble(&$a){ # a is an array of numbers11 12 # length of a13 $m = count($a);14 15 if($m < 2){16 return;17 }18 19 # swap teller20 $n = 0;21 22 # for m numbers, we have m-1 maxes to bubble to the right23 for($i=1; $i<=$m-1; $i++){24 25 # i=1, a max in m numbers to find; 0, m-126 # i=2, a max in m-1 numbers on the left to find; 0, m-2 27 # i=3, a max in m-2 numbers on the left to find; 0, m-3; m-i28 for($j=0; $j<$m-$i; $j++){29 if($a[$j] > $a[$j+1]){30 swap($a[$j], $a[$j+1]);31 $n = 1;32 }33 }34 35 if($n == 0){ # no swap happened, finish the sort36 break; # return37 }38 else{39 $n = 0; # reset teller, continue40 }41 }42 43 return;44 }45 46 $arr = range(5, 0);47 sortBubble($arr);48 echo implode(', ', $arr);49 50 // 0, 1, 2, 3, 4, 551 ?>